Travel Website Php
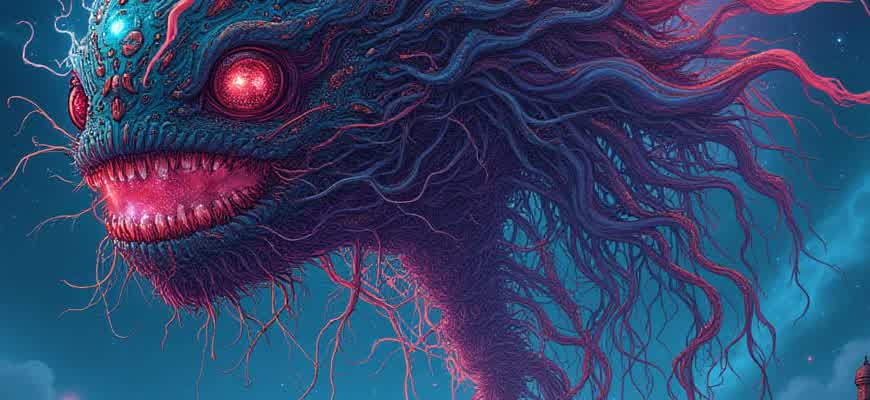
Creating a travel website with PHP offers a robust foundation for managing travel-related data and user interactions. PHP's server-side capabilities allow for seamless integration with databases, providing dynamic content for users based on their preferences. This is especially useful for building personalized travel experiences.
Here are the key features you can implement in a PHP-based travel website:
- User authentication and registration
- Real-time availability checks for flights and hotels
- Booking and payment gateway integration
- Travel destination search and filtering options
PHP provides various frameworks, like Laravel and Symfony, that simplify the development process. These frameworks come with built-in tools to handle routing, templating, and security, allowing developers to focus on building features instead of worrying about common coding tasks.
Important Note: When building a travel site, ensure that your database is optimized for handling large volumes of data, such as flight schedules, hotel availability, and user preferences.
The back-end of the website typically interacts with a relational database, like MySQL, to manage the data. Here's an example of how you might structure a basic table for storing hotel information:
Hotel Name | Location | Price per Night | Rating |
---|---|---|---|
Sunset Beach Resort | Hawaii | $200 | 4.5 |
Mountain View Inn | Colorado | $150 | 4.0 |
How to Build a PHP-Based Travel Website from Scratch
Creating a travel website using PHP can seem like a daunting task, but by breaking down the process into smaller steps, it becomes manageable. The goal is to develop a platform that not only showcases destinations and services but also provides users with an interactive experience. PHP is an excellent choice due to its server-side scripting capabilities and ease of integration with databases like MySQL.
The first step in building your website is setting up the development environment. You’ll need to install a local server like XAMPP or WAMP, which includes Apache, PHP, and MySQL. Once installed, you can start developing and testing your website locally before going live.
Setting Up the Website Structure
When creating the basic structure of your travel website, you need to focus on both the back-end (PHP) and the front-end (HTML, CSS). Below are the main components you’ll need to set up:
- Home page with a destination overview
- Booking system for flights, hotels, and tours
- User registration and login system
- Admin dashboard for managing content and bookings
Make sure your project follows a clear directory structure, such as:
/project_name /assets /css /js /includes /templates /config
Database Setup and Management
For storing user data, bookings, and travel packages, you’ll need a relational database like MySQL. Here is a basic outline of the tables you’ll need:
Table Name | Description |
---|---|
users | Stores user details (name, email, password) |
destinations | Stores information about travel destinations |
bookings | Stores booking information for users |
To set up the database, use the following SQL command:
CREATE DATABASE travel_website; USE travel_website; CREATE TABLE users ( id INT AUTO_INCREMENT PRIMARY KEY, name VARCHAR(100), email VARCHAR(100), password VARCHAR(255) );
Important Considerations
Make sure to sanitize and validate all user inputs to prevent SQL injection and other security issues.
As your project grows, consider adding additional features like a search engine for destinations, integration with payment gateways for bookings, and a recommendation system based on user preferences.
Choosing the Ideal PHP Framework for Your Travel Website
When developing a travel website, selecting the right PHP framework can significantly affect the scalability, maintainability, and performance of your platform. A travel website often requires integration with external APIs, database handling, complex routing, and secure user authentication. The framework you choose should streamline these aspects while providing a robust structure for your application.
There are several PHP frameworks available, each with its own strengths and specific use cases. The key is to align the framework's features with the demands of your project, such as user experience, ease of customization, and support for third-party integrations.
Framework Options for Your Travel Platform
Below are some popular PHP frameworks commonly used for developing travel websites:
- Laravel - Known for its elegant syntax and robust feature set, Laravel is perfect for building complex applications like booking systems and user dashboards.
- Symfony - Ideal for large-scale travel websites that require customizability, Symfony excels in performance and scalability, especially when dealing with high traffic volumes.
- CodeIgniter - If speed and simplicity are a priority, CodeIgniter provides a lightweight option, perfect for smaller travel sites or MVPs.
Factors to Consider When Choosing a Framework
Consider the following when selecting a PHP framework for your project:
- Performance - Opt for a framework that can handle a high number of users and transactions, especially if you're offering booking services in real-time.
- Security - Security is crucial for travel websites. Look for frameworks with built-in protections against SQL injection, cross-site scripting (XSS), and cross-site request forgery (CSRF).
- Community Support - A strong community means more tutorials, documentation, and solutions to common issues.
Comparison Table of PHP Frameworks
Framework | Strengths | Best For |
---|---|---|
Laravel | Elegant syntax, extensive libraries, robust routing | Complex websites with real-time services |
Symfony | Highly customizable, scalable | Enterprise-level travel platforms |
CodeIgniter | Lightweight, fast, simple setup | Small-scale websites or MVPs |
Choosing the right PHP framework for your travel website is essential for optimizing development time, user experience, and long-term maintainability. Make sure to align the framework's features with the specific needs of your project.
Integrating a Booking System into Your Travel Platform with PHP
When building a travel website, one of the key features is the integration of a booking system. This allows users to easily check availability, make reservations, and complete payments. By utilizing PHP, a flexible and widely used server-side scripting language, developers can seamlessly incorporate such systems into their platforms. A booking system can range from simple flight or hotel reservations to more complex multi-day tours or event bookings.
PHP offers various methods for integrating booking functionalities, from using third-party APIs to creating custom solutions. With the help of frameworks like Laravel or Symfony, developers can build secure, scalable, and user-friendly booking systems that can be tailored to specific business needs. Below are some common steps and important considerations when implementing a booking system on your travel site using PHP.
Key Steps to Integrating a Booking System
- Choose a Booking Method: Decide whether to integrate an existing API (such as from a third-party provider) or to build a custom solution from scratch.
- Set Up a Database: Create a database to store information about users, bookings, and payment transactions. PHP works seamlessly with MySQL or PostgreSQL.
- Payment Gateway Integration: Use secure payment gateways (like Stripe or PayPal) to process bookings and payments.
- Design a User Interface: Ensure the booking interface is simple and intuitive for users to select and confirm their reservations.
Integrating a third-party API is often faster but less customizable, while a custom solution allows for more flexibility but requires more time and resources.
Data Flow and Structure Example
Stage | Description |
---|---|
Step 1: User Input | The user selects travel dates, location, and type of service. |
Step 2: Availability Check | The system queries the database or third-party API for available options. |
Step 3: Confirmation | The user confirms the booking and enters payment details. |
Step 4: Payment Processing | The payment is processed, and the booking is confirmed with a receipt sent to the user. |
PHP is a powerful tool for creating dynamic and responsive booking systems that enhance the user experience. Proper integration of such a system can significantly increase the efficiency of your travel website while ensuring a smooth and reliable booking process for your customers.
Improving User Experience for Travel Platforms with PHP
PHP plays a critical role in creating dynamic and interactive travel websites. By leveraging the capabilities of PHP, developers can craft user-centric websites that respond to the needs of modern travelers. The use of efficient server-side processing ensures that users experience fast load times, responsive navigation, and tailored content. Additionally, PHP can seamlessly integrate with databases, enabling personalized trip recommendations and custom itineraries based on user preferences.
When optimizing travel websites, PHP's flexibility allows for easy implementation of features that enhance usability. From real-time booking systems to interactive maps, PHP helps deliver a seamless journey for users from search to booking. By focusing on performance optimization, security measures, and smooth user interfaces, PHP-based solutions can significantly boost overall user satisfaction.
Key Strategies for PHP-Based Travel Site Optimization
- Fast Load Time: Ensure quick page loading by optimizing database queries and using caching mechanisms.
- Mobile Optimization: With the increasing use of mobile devices, make sure the website is fully responsive across all screen sizes.
- User-Focused Navigation: Simplify the user journey with intuitive search filters and a clear, streamlined layout.
Improving website speed and accessibility ensures better engagement and retention, ultimately leading to higher conversion rates on travel booking platforms.
Technical Considerations for Enhanced User Experience
- Database Optimization: Ensure efficient queries and indexing for faster data retrieval.
- Security Measures: Implement strong encryption protocols and secure payment gateways to protect user data.
- Content Personalization: Utilize PHP’s ability to tailor content to user behavior, providing personalized trip suggestions based on browsing history.
Feature | Benefits |
---|---|
Booking Integration | Improves the booking process and ensures real-time availability updates. |
Customizable Itineraries | Enhances the user experience by offering tailored travel plans. |
Managing Travel Content and Packages on PHP Websites
Managing travel content and packages on PHP websites involves handling dynamic data for destinations, itineraries, prices, and booking details. PHP, being a server-side scripting language, is widely used for this purpose due to its ability to integrate with databases and content management systems. By organizing and structuring content effectively, travel websites can offer visitors seamless browsing experiences, making it easier to plan their trips and book services.
The core task is to create and manage various travel packages that cater to different preferences and budgets. A well-structured PHP website should allow for easy addition and modification of travel packages, destinations, and services. This can be achieved through custom-built forms, interactive filters, and a robust database schema that keeps the data organized and easily accessible.
Key Components for Managing Content
- Travel Destination Database: Stores information about locations, activities, and local attractions.
- Package Listings: Contains details about various travel packages, including pricing, duration, and inclusions.
- Booking System: Facilitates online reservations and integrates with payment gateways.
- User Reviews and Ratings: Allows customers to share their experiences, which can be used to improve services.
Important: To ensure smooth content management, using a content management system (CMS) built on PHP, such as WordPress or Joomla, can significantly reduce development time and complexity.
Example: Travel Package Table
Package Name | Destination | Price | Duration |
---|---|---|---|
Beach Getaway | Maldives | $1200 | 7 Days |
Mountain Adventure | Swiss Alps | $1500 | 10 Days |
Cultural Exploration | Japan | $1800 | 14 Days |
To manage travel packages effectively, ensure that the backend system is capable of handling inventory updates in real-time, particularly when dealing with limited availability or special promotions.
Integrating Real-Time Flight and Hotel Information into Your PHP Site
Incorporating real-time flight and hotel booking information into your PHP-based travel website can significantly enhance the user experience. By using APIs from major travel service providers, you can provide dynamic and up-to-date data about flights, accommodations, and availability, directly on your platform. This eliminates the need for users to visit external sites for information, making your platform more attractive and efficient.
To implement this functionality, you’ll need to integrate with external APIs and process the data on your server. These APIs typically offer endpoints for retrieving flight schedules, availability, pricing, and booking options. Below are some important considerations when integrating real-time flight and hotel data into your website.
Key Implementation Steps
- Choose reliable APIs: Select well-documented and trusted APIs like Skyscanner, Amadeus, or Hotelbeds to retrieve real-time flight and hotel data.
- Set up API connections: Utilize PHP's cURL or Guzzle libraries to connect and fetch data from these APIs in real-time.
- Process and display data: Once you retrieve the data, format it into user-friendly results and display it in an organized manner on your website.
Data Integration Example
Here’s a simple table showing how flight information can be structured when fetched from an external API:
Flight | Departure | Arrival | Price |
---|---|---|---|
Flight 101 | New York (JFK) - 8:00 AM | Los Angeles (LAX) - 11:00 AM | $250 |
Flight 202 | Boston (BOS) - 9:00 AM | Chicago (ORD) - 10:45 AM | $180 |
Tip: Consider implementing caching strategies to store frequently requested data temporarily. This will reduce the number of API calls, improve response time, and minimize potential costs associated with API usage.
Hotel Data Integration Example
Similarly, when integrating hotel data, you can display real-time room availability and pricing as follows:
Hotel Name | Location | Availability | Price per Night |
---|---|---|---|
Hotel Central | New York City | Available | $150 |
Seaside Resort | Miami Beach | Fully Booked | N/A |
Securing Customer Data and Transactions on a Travel Website Built with PHP
Ensuring the safety of customer information and transactions is a critical aspect of any travel website, especially one built using PHP. With the growing concerns over cybersecurity, it's essential to implement robust measures to protect sensitive data such as personal details, payment information, and booking history. PHP, being a widely used server-side language, offers several techniques and best practices to mitigate security risks and prevent unauthorized access.
To achieve secure data handling, developers must focus on both securing user data at rest and ensuring the integrity of data during transmission. By incorporating encryption protocols, input validation, and session management, a PHP-based travel site can provide a safe browsing and booking experience for its users.
Key Security Practices for PHP-Based Travel Websites
- Encryption of Sensitive Data: Use SSL/TLS certificates to encrypt data transmitted between the client and server, ensuring that personal and payment details remain confidential.
- Secure Payment Gateways: Integrate trusted payment processors like PayPal or Stripe, which offer advanced security features, including tokenization and fraud detection.
- Input Validation and Sanitization: Properly validate and sanitize user inputs to prevent SQL injection and cross-site scripting (XSS) attacks that can compromise the site’s integrity.
- Session Management: Implement secure session handling techniques, such as regenerating session IDs after login and using secure cookies to prevent session hijacking.
For a travel website, data protection is not just a technical necessity, but also a trust-building element with customers. Failing to safeguard information can lead to severe reputational damage and legal consequences.
Secure Storage of Customer Data
When storing customer information, it is essential to use secure storage practices to ensure data is not compromised in case of a breach. This can include encrypting sensitive data before saving it to the database and regularly updating security protocols to match industry standards.
Storage Method | Security Feature |
---|---|
Database Encryption | Encrypt customer data before storing it to avoid unauthorized access. |
Tokenization | Use tokens instead of actual credit card numbers for payments to minimize exposure to fraud. |
Regular Security Audits | Conduct periodic security audits to identify vulnerabilities and ensure ongoing protection. |
Scaling Your PHP Travel Website for Growing Traffic and Bookings
As your travel website gains popularity, handling an increasing volume of traffic and bookings becomes a critical concern. Effective scaling is essential for maintaining website performance and ensuring that users have a seamless experience, especially during peak times. PHP-based websites can leverage various strategies to efficiently manage larger loads without compromising functionality or speed.
Optimizing both server resources and code can significantly improve the ability of your travel website to scale. With growing numbers of users making bookings and browsing travel options, addressing potential bottlenecks becomes crucial. Here are some effective strategies for scaling your PHP website:
Key Strategies for Scaling PHP Websites
- Load Balancing: Distribute traffic evenly across multiple servers to avoid overloading a single machine and to ensure continuous availability.
- Database Optimization: Optimize queries and implement indexing to improve database performance. Consider using NoSQL for certain types of data.
- Caching: Implement caching mechanisms (e.g., Redis, Memcached) to reduce the load on the server and speed up page rendering for users.
- Content Delivery Network (CDN): Use a CDN to serve static resources like images and CSS, reducing server load and improving load times.
Monitoring and Maintaining Performance
Regularly monitoring your server’s health and website performance is vital to preemptively identify and resolve issues before they affect user experience.
- Performance Testing: Use tools like Apache JMeter or New Relic to simulate traffic and detect performance bottlenecks.
- Scalable Infrastructure: Implement cloud hosting services such as AWS or Google Cloud, which can automatically adjust resources based on traffic demands.
- Database Sharding: Split your database into smaller, more manageable parts to reduce query load and improve scalability.
Technical Considerations
Technique | Description | Benefits |
---|---|---|
Load Balancing | Distributes traffic across multiple servers to ensure high availability. | Improved performance, reduced downtime, better fault tolerance. |
Database Caching | Caches frequent queries to minimize database access time. | Faster response time, reduced server load, improved scalability. |
Cloud Hosting | Scales server resources dynamically based on real-time traffic. | Cost-effective, highly scalable, reduces manual intervention. |