Route Optimization in R
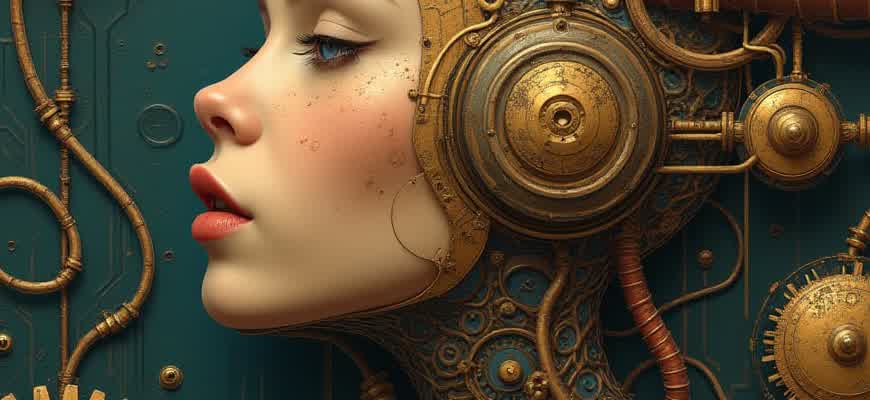
Route optimization is a critical aspect of logistics and transportation management, aiming to minimize travel time, fuel consumption, and operational costs. R, a powerful statistical programming language, provides a range of tools to efficiently solve routing problems by analyzing geographical data and applying optimization algorithms.
Key steps in route optimization with R include:
- Data preparation: Geographical coordinates and route constraints must be collected and formatted.
- Model selection: Appropriate algorithms like the Traveling Salesman Problem (TSP) or Vehicle Routing Problem (VRP) are chosen based on the specific scenario.
- Optimization: Using R packages like ggmap or igraph, the best possible routes are identified.
“Optimization models in R allow companies to significantly reduce logistical expenses and improve delivery efficiency.”
Example of a route optimization solution in R:
City | Latitude | Longitude |
---|---|---|
New York | 40.7128 | -74.0060 |
Los Angeles | 34.0522 | -118.2437 |
Chicago | 41.8781 | -87.6298 |
Setting Up R Libraries for Route Optimization Tasks
To perform route optimization in R, it is essential to first install and load the right libraries that provide the necessary algorithms and data manipulation functions. The libraries typically used for routing tasks include those for distance matrix computation, optimization models, and geographic data processing.
Before diving into route optimization, ensure that you have installed the required packages. This setup will help you leverage the full capabilities of R in managing and solving routing problems efficiently.
Common R Libraries for Route Optimization
- ggplot2: For visualizing routes and geographic data.
- igraph: Used for graph-based route planning and optimization tasks.
- gdist: For calculating geographic distances between locations.
- distillery: Helps in solving various optimization problems, including route planning.
- ROCR: Useful for evaluating the performance of optimization models.
Installing and Loading Libraries
- Install the required libraries using the
install.packages()
function. - Load each library into your R environment with
library()
. - Ensure that each library is compatible with your R version to avoid conflicts during execution.
Important: It’s a good practice to keep your libraries up to date to access the latest features and improvements in route optimization tasks.
Example of Library Setup
Library | Purpose | Installation Command |
---|---|---|
ggplot2 | Data visualization for routes | install.packages("ggplot2") |
igraph | Graph-based optimization | install.packages("igraph") |
gdist | Distance calculations | install.packages("gdist") |
Handling Geographic Data for Route Planning
Effective route planning relies heavily on the quality and structure of geographic data. This data typically includes geographic coordinates, road networks, and other locational attributes essential for calculating optimal paths. When working with such data in R, it’s crucial to manage it efficiently to ensure accurate and precise results. The primary components of geographic data include points (locations), lines (roads, streets), and polygons (areas of interest such as cities or zones). These elements need to be properly represented using suitable data structures like spatial objects or geographic data frames in R.
R provides various packages for handling geographic data, such as 'sf' (simple features) and 'sp'. These packages enable users to work with spatial data in a way that is both scalable and efficient. With these tools, one can easily import, manipulate, and analyze geographic data to determine optimal routes based on distance, time, or other criteria. Geographic data is often visualized in maps, which can be further analyzed for route optimization purposes.
Types of Geographic Data
- Points: Locations represented by latitude and longitude coordinates (e.g., delivery addresses).
- Lines: Roads or paths between two or more points (e.g., streets, highways).
- Polygons: Enclosed areas representing boundaries (e.g., city limits, delivery zones).
Common Tools in R for Handling Geographic Data
- sf Package: A simple and effective way to handle spatial data, including vector data formats like points, lines, and polygons.
- sp Package: A traditional package for spatial data manipulation, offering a wide range of functions for geographic analysis.
- leaflet: Used for creating interactive maps, allowing users to visualize geographic data during route planning.
Important: Before starting any route optimization task, ensure your geographic data is cleaned and accurately reflects the real-world environment to avoid errors in planning.
Data Structure Example
Data Type | Example |
---|---|
Point | Lat: 40.7128, Long: -74.0060 (New York City) |
Line | Path between two points (e.g., Road A to Road B) |
Polygon | Area bounded by coordinates (e.g., City of New York) |
Implementing Shortest Path Algorithms in R
Shortest path algorithms are essential in route optimization, where the goal is to determine the quickest route between two points in a graph. In R, various libraries can be used to implement these algorithms, such as the 'igraph' package. This package offers functionality for both directed and undirected graphs, making it highly suitable for applications in transportation, logistics, and network analysis.
R provides several methods for solving shortest path problems, each designed to handle specific types of graphs and optimization needs. The two most commonly used algorithms are Dijkstra’s algorithm and Bellman-Ford algorithm. While both are used for finding the shortest path in weighted graphs, Dijkstra’s algorithm performs more efficiently on graphs with non-negative edge weights, whereas Bellman-Ford can handle negative edge weights and detect negative cycles.
Key Steps in Implementing Shortest Path Algorithms
- Load necessary libraries such as 'igraph' or 'ggraph'.
- Create a graph object that represents the nodes and edges of your network.
- Use the appropriate algorithm based on the characteristics of your graph.
- Visualize the results to verify the computed shortest paths.
Common Algorithms
- Dijkstra's Algorithm: Efficient for graphs with non-negative weights. It uses a greedy approach to explore the shortest path incrementally.
- Bellman-Ford Algorithm: Handles negative weights and can detect negative weight cycles.
- A* Algorithm: An improvement over Dijkstra, which includes a heuristic to speed up the search.
Example Code for Dijkstra's Algorithm
library(igraph)
# Create a graph
g <- graph_from_edgelist(cbind(c(1,1,2,3,4,4), c(2,3,4,4,5,5)), directed=FALSE)
E(g)$weight <- c(10, 20, 30, 10, 40, 50)
# Compute shortest path using Dijkstra's algorithm
shortest_path <- shortest_paths(g, from=1, to=5, weights=E(g)$weight)
shortest_path
Important Considerations
When implementing shortest path algorithms in R, it is crucial to ensure that the graph is correctly defined, particularly in terms of edge weights. Incorrect weight assignments or graph representations can lead to erroneous results.
Example Output
Start Node | End Node | Path | Path Length |
---|---|---|---|
1 | 5 | 1-4-5 | 60 |
Integrating Real-Time Traffic Data for Dynamic Routing
Dynamic routing has become an essential component in optimizing travel times, especially in urban environments. By using real-time traffic information, routes can be adjusted based on current road conditions such as congestion, accidents, and construction activities. This approach helps minimize travel time and enhance efficiency, which is crucial for both logistics and everyday commuting.
Real-time traffic data provides a constantly updated view of road conditions, allowing for the integration of live data feeds into route planning algorithms. These data sources can include GPS information, traffic sensors, and social media reports, offering valuable insights for adjusting routes in real-time.
Benefits of Real-Time Traffic Integration
- Reduced Travel Time: Dynamically adjusted routes lead to quicker travel by avoiding congested areas.
- Improved Efficiency: Real-time updates enable more effective routing, saving fuel and resources.
- Better User Experience: Drivers or logistics managers are guided through the most efficient paths, minimizing delays.
Challenges to Overcome
- Data Accuracy: The quality and reliability of traffic data must be high to make valid route decisions.
- Real-Time Processing: Integrating and processing large volumes of data in real-time can be computationally intensive.
- System Compatibility: Real-time data needs to be compatible with existing routing algorithms and systems.
Integrating real-time traffic data allows for more informed decision-making in routing algorithms, enhancing the overall efficiency and effectiveness of travel planning systems.
Data Flow Example
Source | Data Type | Usage |
---|---|---|
GPS Devices | Location Data | Track current vehicle position and route adjustments. |
Traffic Sensors | Congestion Levels | Assess real-time traffic conditions along different routes. |
Social Media | Accident/Incident Reports | Provide updates on accidents or unexpected roadblocks. |
Using R for Fleet Management and Route Scheduling
Fleet management and route scheduling are critical elements for businesses relying on transportation. By leveraging R, companies can optimize the movement of vehicles, reduce operational costs, and improve efficiency. R’s diverse ecosystem of packages and libraries offers the tools necessary for implementing complex algorithms and models to manage fleet operations effectively.
In particular, R enables the analysis of large datasets to determine optimal routes, manage vehicle allocation, and predict maintenance schedules. R provides methods to calculate distance, time, and cost factors for different routes, ensuring an efficient deployment of resources. By automating these processes, businesses can enhance decision-making and operational performance.
Key R Tools for Route Optimization
- Geo-spatial Libraries: R packages like sf and sp help in working with geographic data, essential for route planning and optimization.
- Optimization Algorithms: Packages like lpSolve and ompr provide linear programming tools to solve route scheduling problems.
- Data Handling: Tools such as dplyr and tidyr are invaluable for cleaning, transforming, and managing transportation datasets.
Steps for Implementing Fleet Management in R
- Data Collection: Gather data on vehicle locations, traffic conditions, and route preferences.
- Data Preprocessing: Clean and transform the data to ensure its usability in optimization models.
- Route Optimization: Use optimization algorithms to find the shortest or most cost-effective routes based on your data.
- Fleet Allocation: Assign vehicles to routes, considering factors like load capacity, fuel consumption, and time constraints.
- Monitoring: Track vehicle performance and adjust routes in real-time based on updated traffic and operational data.
Example of Route Optimization Results
Route | Distance (km) | Time (hrs) | Cost ($) |
---|---|---|---|
Route 1 | 150 | 2.5 | 120 |
Route 2 | 120 | 2 | 100 |
Route 3 | 180 | 3 | 140 |
By analyzing and comparing various routes, businesses can select the most efficient paths, thereby reducing fuel costs and improving delivery times.
Optimizing Routes for Multiple Stops with R
When dealing with route optimization tasks involving multiple stops, it's essential to find the most efficient path that minimizes travel time or distance. This problem is often approached using algorithms such as the Traveling Salesman Problem (TSP) or vehicle routing problem (VRP), which can be solved using various packages available in R. With the right tools, you can automate the process of determining the optimal routes for delivery, logistics, or sales visits, ultimately saving time and resources.
R provides a wide array of libraries such as “TSP” and “vehicleRouting” that allow you to model and solve multi-stop optimization problems. These libraries offer different techniques for minimizing travel distance and improving efficiency, especially when you have a large set of locations to cover. Additionally, the use of visualization tools can help present the optimized routes clearly for decision-making.
Steps to Optimize Routes
- Data Collection: Gather all locations (coordinates or addresses) that need to be visited. This data will serve as input for your optimization model.
- Preprocessing: Convert the raw data into a usable format, such as a distance matrix, that calculates the travel cost between each pair of locations.
- Modeling: Use R's built-in functions to define the optimization model, typically based on TSP or VRP algorithms.
- Solution Evaluation: After obtaining the optimal route, evaluate its efficiency and adjust parameters if necessary to improve the solution.
Example of R Packages for Route Optimization
Package | Description | Key Features |
---|---|---|
TSP | Solves the Traveling Salesman Problem. | Offers exact and heuristic algorithms, supports distance matrices. |
vehicleRouting | Helps with vehicle routing problems. | Optimizes multiple routes and includes constraints like capacity. |
"The TSP package in R provides a range of powerful functions to solve routing problems quickly and efficiently, making it a vital tool for logistics professionals."
Visualizing Route Optimization Results in R
Effective visualization of route optimization outcomes is essential for interpreting the efficiency and feasibility of different routes. In R, there are several packages and methods that allow you to visualize optimized paths, ensuring that stakeholders can easily understand the results. A popular approach is to use geographic maps to plot routes, providing a clear view of the optimized journey. This is typically achieved with packages such as ggplot2 or leaflet, which allow the creation of interactive maps or static route plots. These maps can highlight the optimized route, showing the sequence of waypoints and the calculated distance.
Another useful way to visualize results is through network diagrams. These can be particularly helpful when dealing with multiple routes or nodes in a large network. By representing each location as a node and the possible paths as edges, it’s easier to compare different routing strategies. Using R’s igraph package, you can create a graph that visually represents the connections between various locations, allowing for clear assessment of the most efficient path.
Key Methods for Visualization
- Interactive maps using the leaflet package.
- Static route plots via ggplot2.
- Network diagrams with the igraph package.
Steps for Plotting Optimized Routes
- Install and load the necessary R packages.
- Prepare your data, ensuring that the locations are properly geocoded.
- Use routing algorithms to compute the optimal route.
- Plot the results using either static or interactive map functions.
- Refine the visualization by customizing labels, colors, and markers.
Tip: For large-scale route optimizations, consider using leaflet for interactive maps to allow users to zoom in and explore different routes in detail.
Example of Route Data
Location | Latitude | Longitude |
---|---|---|
Start Point | 40.748817 | -73.985428 |
Waypoint 1 | 40.749822 | -73.977031 |
Waypoint 2 | 40.750675 | -73.970560 |
End Point | 40.751645 | -73.965348 |